Quote: "I'll have to finish the rest later, I gotta get something to eat."

Must be the funniest thing I have ever heard from you! Enjoy your meal
I think I am starting to understand, thanks a lot for your efforts!
TheComet
EDIT: I can not figure this out. Here is the test code I'm using(I know, very messy):
rem setup screen
set display mode 1280,800,32
sync off
backdrop off
color backdrop 0
rem set variables
systems=3
max_waypoints=3
max_selections=3
size#=(20*((systems+1)*(max_waypoints+1)*(max_selections+1)))+4
array_size=(4*((systems+1)*(max_waypoints+1)*(max_selections+1)))+4
rem make the memblock and arrays
make memblock 1,size#
dim yeah1#(3,3,3)
dim yeah2#(3,3,3)
dim yeah3#(3,3,3)
dim yeah4#(3,3,3)
rem write some random numbers to memblock and arrays
for x=1 to 3
for y=1 to 3
for z=1 to 3
numb1#=rnd(1000)/300
numb2#=rnd(1000)/300
numb3#=rnd(1000)/300
numb4#=rnd(1000)/300
position=(array_size*0)+(4*((x+1)*(y+1)*(z+1)))+4:write memblock float 1,position,numb1#
position=(array_size*1)+(4*((x+1)*(y+1)*(z+1)))+4:write memblock float 1,position,numb2#
position=(array_size*2)+(4*((x+1)*(y+1)*(z+1)))+4:write memblock float 1,position,numb3#
position=(array_size*3)+(4*((x+1)*(y+1)*(z+1)))+4:write memblock float 1,position,numb4#
yeah1#(x,y,z)=numb1#
yeah2#(x,y,z)=numb2#
yeah3#(x,y,z)=numb3#
yeah4#(x,y,z)=numb4#
next z
next y
next x
rem set text position
posx=0
posy=-1
rem print variables. They do not match?!
for x=1 to 3
for y=1 to 3
for z=1 to 3
inc posy:if posy>64 then posy=0:inc posx,50
position=(array_size*0)+(4*((x+1)*(y+1)*(z+1)))+4:text posx,posy*12,str$(memblock float(1,position))+","+str$(yeah1#(x,y,z))
inc posy:if posy>64 then posy=0:inc posx,50
position=(array_size*1)+(4*((x+1)*(y+1)*(z+1)))+4:text posx,posy*12,str$(memblock float(1,position))+","+str$(yeah2#(x,y,z))
inc posy:if posy>64 then posy=0:inc posx,50
position=(array_size*2)+(4*((x+1)*(y+1)*(z+1)))+4:text posx,posy*12,str$(memblock float(1,position))+","+str$(yeah3#(x,y,z))
inc posy:if posy>64 then posy=0:inc posx,50
position=(array_size*3)+(4*((x+1)*(y+1)*(z+1)))+4:text posx,posy*12,str$(memblock float(1,position))+","+str$(yeah4#(x,y,z))
next z
next y
next x
rem end
wait key
end
What I am doing is giving four 3-dimensional arrays random numbers, and writing the same info to a memblock, so the four arrays are in the memblock. But when I read them again they are not the same at all. When you see them print to the screen, the two numbers separated by a comma have to be the same. But they aren't. Am I writing them incorrectly?
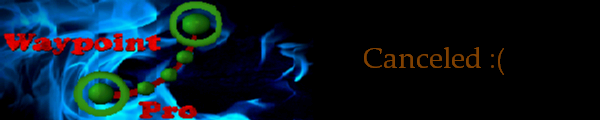
Make the path of your enemies easier with Waypoint Pro!