@Spectre 117:
Here is your code with smoothness added. I used the
curvevalue command, which smoothly moves one number to another. Basically what the code does is record the camera's current position, wherever it may be. Then it puts the camera where it's supposed to be (behind the object) and records that position. Then, using the curvevalue command, it smoothly transitions the camera's coordinates from the current coordinates to the coordinates it's supposed to be at.
Curveangle does the same thing as
curvevalue, only it does it for angles. The code then records these "transitioning" coordinates in some variables and then puts the camera there.
NOTE: Because of some weird rotating issue, the PITCH CAMERA UP/DOWN commands didn't work with smoothing (there was some weird sudden jerkiness). So I changed them to use
xrotate camera instead. Pitching the camera up by 90 degrees is the same as subtracting 90 degrees from the camera's current x angle. Pitching it down is the same as adding 90 degrees. Keep in mind that the TURN OBJECT LEFT/RIGHT commands may also cause similar problems. This can be fixed by using the
yrotate object command instead.
Anyway, here's the modified code with smoothing. It's commented to make it easier to understand. The variable names are also really long to make things easier
`Record the camera's position before you put it where you want it.
WhereItWasBeforeX#=camera position x()
WhereItWasBeforeY#=camera position y()
WhereItWasBeforeZ#=camera position z()
`Record the camera's angle before you put it where you want it. The Wrapvalue() command just keeps the angle measure between 0 and 360 degrees. For example, 365 degrees would become 5 degrees, since they're both the same thing.
WhereItWasBeforeAngleX#=Wrapvalue( camera angle x() )
WhereItWasBeforeAngleY#=Wrapvalue( camera angle y() )
WhereItWasBeforeAngleZ#=Wrapvalue( camera angle z() )
`Put the camera where you want it to be.
position camera object POSITION X(13), OBJECT POSITION Y(13), OBJECT POSITION Z(13)
SET CAMERA TO OBJECT ORIENTATION 13
move camera -20
xrotate camera camera angle x()-90
move camera 7
xrotate camera camera angle x()+90
`Record this new position (where you WANT the camera to be) in some variables
WhereItShouldBeX#=camera position x()
WhereItShouldBeY#=camera position y()
WhereItShouldBeZ#=camera position z()
`Record the new angle in some variables
WhereItShouldBeAngleX#=Wrapvalue( camera angle x() )
WhereItShouldBeAngleY#=Wrapvalue( camera angle y() )
WhereItShouldBeAngleZ#=Wrapvalue( camera angle z() )
`Smoothly transition the camera from where it was before to where you want it to be. Record this "transitioned value" in new variables.
Smoothness#=5.0 `This is how much smoothness you want. 1 is no smoothness and higher numbers give you more and more smoothness.
`(The curvevalue command basically moves a value from one value to another within a certain number of steps. The more steps, the longer it takes for the value to get where you want it to be.
cx#=Curvevalue(WhereItShouldBeX#,WhereItWasBeforeX#,Smoothness#)
cy#=Curvevalue(WhereItShouldBeY#,WhereItWasBeforeY#,Smoothness#)
cz#=Curvevalue(WhereItShouldBeZ#,WhereItWasBeforeZ#,Smoothness#)
`Smoothly transition the camera's angle. I will use the Curveangle command here because it's better for angles than Curvevalue.
cax#=Curveangle(WhereItShouldBeAngleX#,WhereItWasBeforeAngleX#,Smoothness#)
cay#=Curveangle(WhereItShouldBeAngleY#,WhereItWasBeforeAngleY#,Smoothness#)
caz#=Curveangle(WhereItShouldBeAngleZ#,WhereItWasBeforeAngleZ#,Smoothness#)
`Finally, put the camera in its place and rotate it based on the smooth transitions above.
position camera cx#,cy#,cz#
rotate camera cax#,cay#,caz#
I hope this helps
EDIT: Or you could just use SET CAMERA TO FOLLOW for smoothness as someone mentioned earlier.
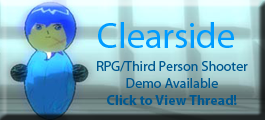
Guns, cinematics, stealth, items and more!