While we are on this subject there is a way to greatly increase your performance if you plan to use tile-based maps. The objective is to draw the tile map as few times as possible by drawing it once into a BITMAP(drawing surface, not the .BMP format) and copying it over to the screen. You may also save the drawn tile map into an image and paste that too but Bitmap copying is so much faster than image grabbing.
Here's another fast tutorial:
1. Lets make an example terrain tileset to use in this example:
sync on
sync rate 0
DXS INITIALIZE
for lpy = 0 to 9
for lpx = 0 to 9
Grn = (lpx+lpy*10)*2.5
ink rgb(0,Grn,0),0
box lpx*16,lpy*16,lpx*16+16,lpy*16+16
next lpx
next lpy
get image 1,0,0,160,160,1
do
paste image 1,100,100
sync
cls
loop
2. Now we make an Advanced Sprite of the tileset 10x10 tiles and draw a single random frame to a random coordinate on the screen:
sync on
sync rate 0
DXS INITIALIZE
for lpy = 0 to 9
for lpx = 0 to 9
Grn = (lpx+lpy*10)*2.5
ink rgb(0,Grn,0),0
box lpx*16,lpy*16,lpx*16+16,lpy*16+16
next lpx
next lpy
get image 1,0,0,160,160,1
SpritePack = DXS CREATE SPRITE PACK()
SpriteTerrain = DXS CREATE SPRITE FROM IMAGE(1)
DXS ADD SPRITE TO PACK SpriteTerrain,SpritePack
DXS SET SPRITE FRAMESET SpriteTerrain,10,10
set text opaque
do
DXS BEGIN SPRITE PACK RENDER SpritePack
DXS USE FILTER 0
DXS SET SPRITE FRAME SpriteTerrain,rnd(99)+1
DXS DRAW SPRITE FRAME SpriteTerrain,rnd(640),rnd(480)
DXS END SPRITE PACK RENDER SpritePack
text 0,0,"fps: "+str$(screen fps())
sync
`cls
loop
3. Next we make a terrain array and fill the screen with the map:
sync on
sync rate 0
DXS INITIALIZE
for lpy = 0 to 9
for lpx = 0 to 9
Grn = (lpx+lpy*10)*2.5
ink rgb(0,Grn,0),0
box lpx*16,lpy*16,lpx*16+16,lpy*16+16
next lpx
next lpy
get image 1,0,0,160,160,1
SpritePack = DXS CREATE SPRITE PACK()
SpriteTerrain = DXS CREATE SPRITE FROM IMAGE(1)
DXS ADD SPRITE TO PACK SpriteTerrain,SpritePack
DXS SET SPRITE FRAMESET SpriteTerrain,10,10
set text opaque
MapX = 40
MapY = 30
dim MapTerrain(MapX,MapY)
For lpy = 0 to MapY
for lpx = 0 to MapX
MapTerrain(lpx,lpy) = rnd(99)+1
next lpx
next lpy
do
gosub DrawTerrain
text 0,0,"fps: "+str$(screen fps())
sync
`cls
loop
DrawTerrain:
DXS BEGIN SPRITE PACK RENDER SpritePack
DXS USE FILTER 0
for lpy = 0 to MapY
for lpx = 0 to MapX
DXS SET SPRITE FRAME SpriteTerrain,MapTerrain(lpx,lpy)
DXS DRAW SPRITE FRAME SpriteTerrain,lpx*16,lpy*16
next lpx
next lpy
DXS END SPRITE PACK RENDER SpritePack
return
The last example still has a fast fps rate because it was only drawing 1 sprite each loop but notice when you are now drawing 40x30=1200 sprites per loop, the fps drops greatly!
4. To speed this up we create a bitmap, draw the terrain on it once and copy it over every loop instead of drawing all 1200 sprites:
sync on
sync rate 0
DXS INITIALIZE
for lpy = 0 to 9
for lpx = 0 to 9
Grn = (lpx+lpy*10)*2.5
ink rgb(0,Grn,0),0
box lpx*16,lpy*16,lpx*16+16,lpy*16+16
next lpx
next lpy
get image 1,0,0,160,160,1
SpritePack = DXS CREATE SPRITE PACK()
SpriteTerrain = DXS CREATE SPRITE FROM IMAGE(1)
DXS ADD SPRITE TO PACK SpriteTerrain,SpritePack
DXS SET SPRITE FRAMESET SpriteTerrain,10,10
set text opaque
MapX = 40
MapY = 30
dim MapTerrain(MapX,MapY)
For lpy = 0 to MapY
for lpx = 0 to MapX
MapTerrain(lpx,lpy) = rnd(99)+1
next lpx
next lpy
create bitmap 1,640,480 REM Creates a new drawing surface with the same size as the screen
set current bitmap 0 REM Bitmap 0 is the screen.
do
gosub DrawTerrain
text 0,0,"fps: "+str$(screen fps())
sync
`cls
loop
DrawTerrain:
if DrawTerrainFlag = 0
DrawTerrainFlag = 1 REM Flag, manually set flag to 0 to redraw terrain onto bitmap 1
set current bitmap 1 REM Switch drawing operation to Bitmap 1
DXS BEGIN SPRITE PACK RENDER SpritePack
DXS USE FILTER 0
for lpy = 0 to MapY
for lpx = 0 to MapX
DXS SET SPRITE FRAME SpriteTerrain,MapTerrain(lpx,lpy)
DXS DRAW SPRITE FRAME SpriteTerrain,lpx*16,lpy*16
next lpx
next lpy
DXS END SPRITE PACK RENDER SpritePack
set current bitmap 0 REM Switch drawing operation back to the screen
endif
copy bitmap 1,0,0,640,480, 0,0,0,640,480 REM Best if you F1 this.
return
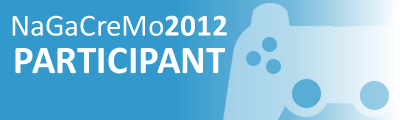